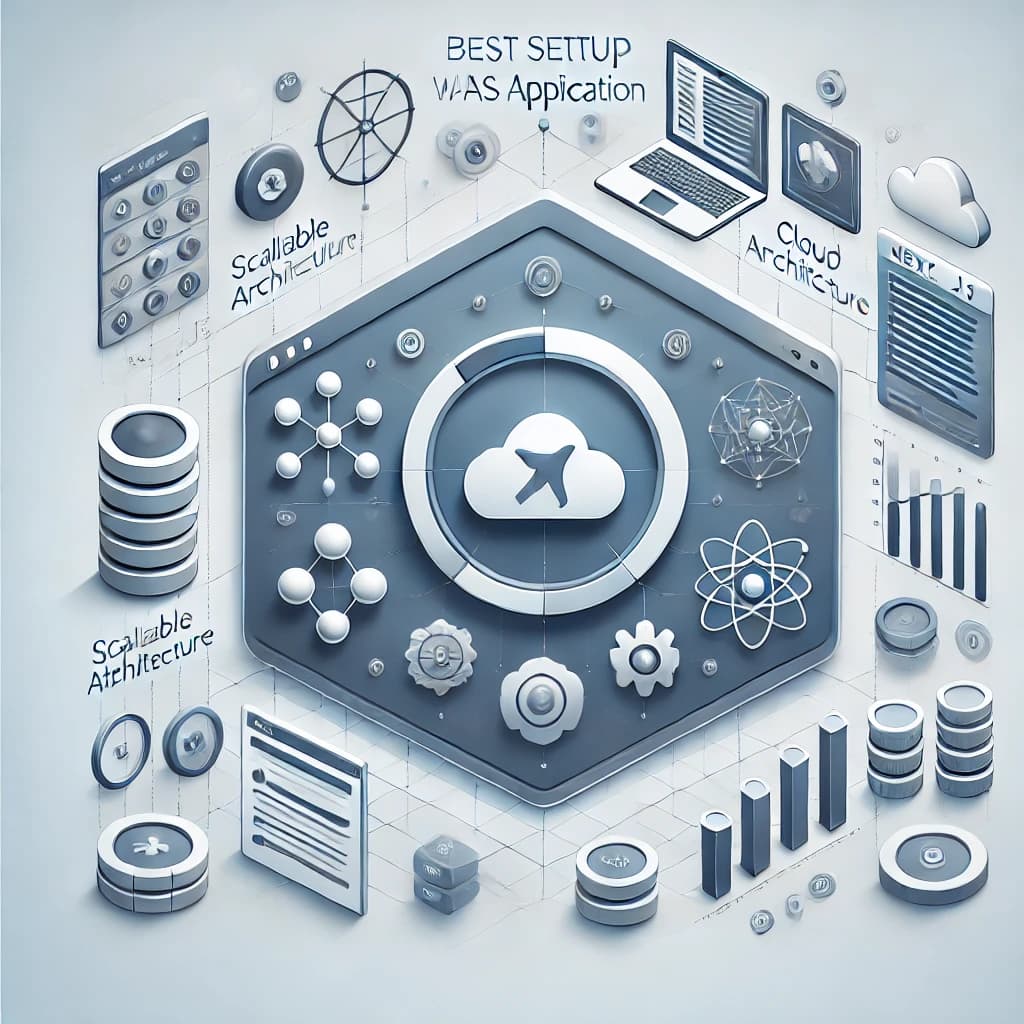
SaaS in Next.js: Best Modern Setup for 2024
Bohdan / October 28, 2024
SaaS in Next.js: Best Modern Setup for 2024
Learn how to develop a powerful SaaS application using Next.js, focusing on scalable architecture, efficient API handling, security best practices, and deployment strategies to meet the demands of modern web applications in 2024.
Table of Contents
- Introduction
- Why Next.js for SaaS
- SaaS Architecture Fundamentals
- Data Layer and Database Choices
- API Layer with GraphQL and REST
- Authentication and Authorization Solutions
- UI and UX Best Practices
- Security Considerations
- Performance and Optimization Techniques
- Deployment and Scaling Strategies
- Feature Comparison Table
- Conclusion
Introduction
The SaaS market is highly competitive, and delivering a scalable, secure, and responsive application is essential for user retention and satisfaction. Next.js offers a modern, flexible framework optimized for both SSR (server-side rendering) and API integrations, making it a top choice for developing SaaS products.
Why Next.js for SaaS
Next.js provides a powerful foundation for SaaS applications with its flexible rendering options, file-based routing, and API routes. The combination of these features allows for efficient data handling, seamless navigation, and high-performance user interfaces that are critical for SaaS applications.
Key Advantages of Next.js for SaaS
- Full-stack Capabilities: Next.js supports both frontend and backend logic, enabling efficient API handling and integration.
- Static Generation & SSR: Improved SEO and performance.
- Optimized for Performance: Built-in optimizations like automatic code-splitting and lazy loading.
- Serverless & Edge Support: Easily deploy serverless functions for scalable backend infrastructure.
- Easy Integration with Tools: Compatible with databases, authentication services, and deployment platforms.
SaaS Architecture Fundamentals
A successful SaaS architecture in 2024 requires a modular, scalable setup that prioritizes security and performance.
Core SaaS Architecture Components
- Next.js for UI and server-side functions.
- GraphQL or REST APIs to connect with databases and manage data efficiently.
- Serverless Functions (e.g., Vercel Functions, AWS Lambda) for a scalable backend.
- Database (PostgreSQL, MongoDB, or PlanetScale) managed by reliable providers.
- Authentication via services like Auth0 or NextAuth, incorporating role-based access for a secure multi-tenant structure.
Folder Structure for Maintainability
Organizing your project files is essential for scalability and collaboration.
├── /pages │ ├── /api │ ├── index.js ├── /components ├── /lib ├── /hooks ├── /services ├── /styles ├── next.config.js └── README.md
python
Data Layer and Database Choices
For data handling in a SaaS setup, choose a scalable database and a data-access strategy that supports complex queries and relationships.
Recommended Databases
- PostgreSQL with Prisma ORM for relational data management.
- MongoDB for document-based storage, ideal for high flexibility.
- PlanetScale (MySQL-compatible, serverless) for a scalable cloud-based solution.
API Layer with GraphQL and REST
The API layer is the backbone of data communication in a SaaS app. While REST APIs work well for simple applications, GraphQL offers a more flexible, efficient data-fetching model.
Example of GraphQL with Next.js
js
import { gql } from '@apollo/client';
import client from '../lib/apollo-client';
export async function getData() {
const { data } = await client.query({
query: gql`
query {
getItems {
id
name
description
}
}
`,
});
return data;
}
Authentication and Authorization Solutions
For SaaS, secure and scalable authentication is critical. NextAuth and Auth0 are popular choices, providing robust multi-tenant support and role-based access. Implementing NextAuth for Custom Roles
js
import NextAuth from 'next-auth';
import Providers from 'next-auth/providers';
export default NextAuth({
providers: [
Providers.Google({
clientId: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET,
}),
],
callbacks: {
async session(session, user) {
session.user.role = user.role; // Add role to session
return session;
},
},
});
UI and UX Best Practices
For SaaS, the user interface must be intuitive and performant. Next.js offers features like static generation, lazy loading, and image optimization, which are essential for delivering a high-quality user experience.
Modular Components: Break down UI elements into reusable, maintainable components. State Management: Use libraries like Redux or Zustand for managing global state. Responsive Design: Ensure the app is usable on all screen sizes. Optimized Images: Use Next.js’s component for faster loading.
Security Considerations
Security is paramount for SaaS. Consider these best practices:
Data Validation and Sanitization: Prevent SQL injection and XSS attacks. Rate Limiting and Throttling: Protect your APIs from abuse. Environment Variables: Secure sensitive information using .env and services like AWS Secrets Manager. SSL/TLS: Ensure secure data transmission.
Performance and Optimization Techniques
Performance is key for SaaS, and Next.js offers several built-in optimizations.
Image Optimization: Use Next.js component for optimized images. Code Splitting: Load code only when necessary, reducing initial load times. CDN Integration: Use a CDN like Cloudflare for faster asset delivery. Database Caching and Indexing: Improve database response times.
Deployment and Scaling Strategies
Deploying and scaling a SaaS application requires a robust infrastructure that can handle increased demand. Suggested Deployment Workflow
CI/CD: Set up continuous integration and deployment with GitHub Actions or CircleCI. Serverless Backend: Deploy backend logic as serverless functions to handle scaling. CDN and Edge Functions: Use a global CDN and edge functions for low-latency delivery.
Feature Comparison Table
Below is a comparison of popular tools and practices for SaaS development with Next.js.
Feature | Next.js | Auth0 | Supabase | Prisma | Vercel |
---|---|---|---|---|---|
Authentication | Built-in support | OAuth & RBAC | Integrated Auth | Custom roles | SSO ready |
Database | Custom API routes | Not applicable | PostgreSQL & more | ORM for various DBs | Managed with serverless |
Performance | SSR & ISR | Managed sessions | Database caching | Optimized queries | Global edge network |
Deployment | Vercel optimized | Easy integration | Serverless DB | Cloud-ready ORM | Seamless scaling |
Ideal For | SaaS & e-commerce | Multi-tenant SaaS | Data-rich SaaS | Database management | Global applications |
Conclusion
Next.js, with its robust architecture and modern tooling, provides a powerful platform for SaaS development in 2024. By leveraging modular architecture, optimized APIs, and best-in-class security practices, you can deliver a scalable and high-performance SaaS product that meets today’s demanding standards. Take advantage of Next.js’s features to maximize efficiency, and choose your tools carefully to build a solution that can grow with your business.
Happy building!