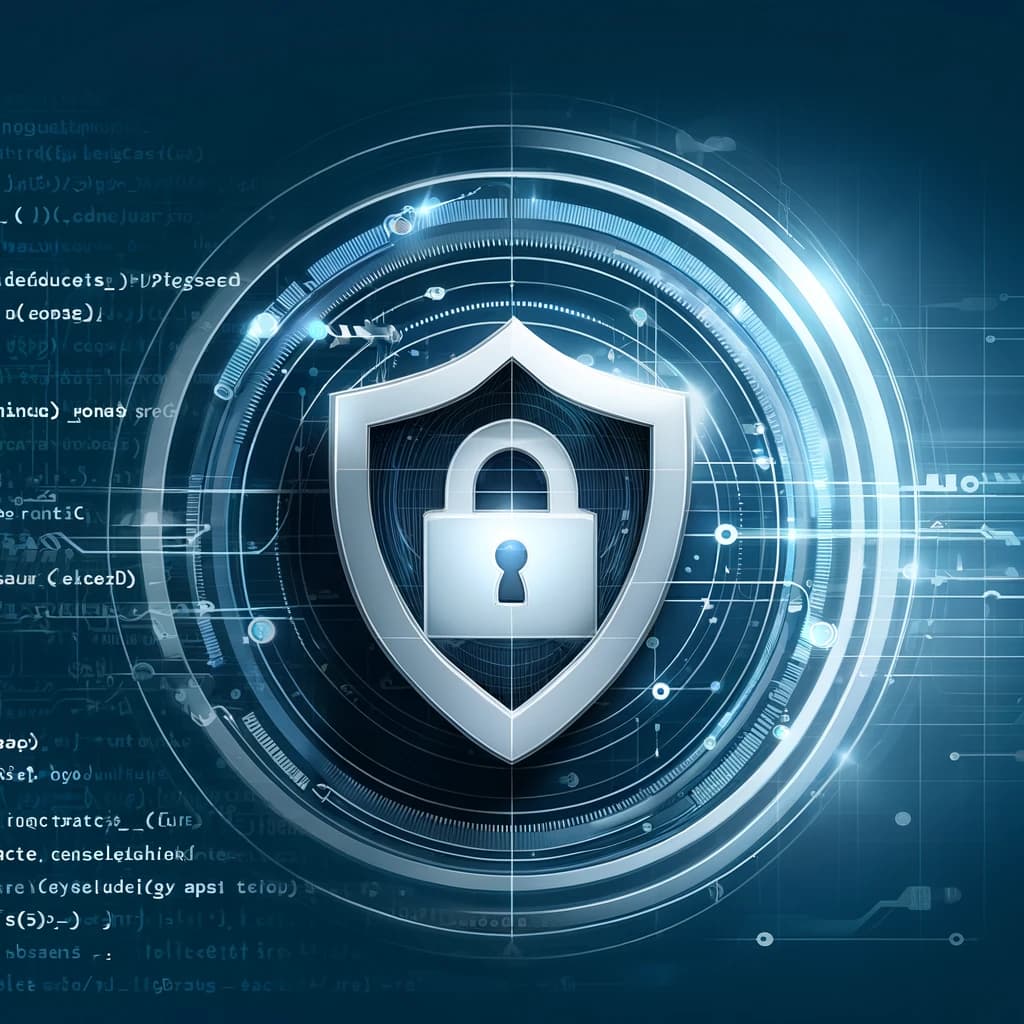
Mastering Security in PHP: Best Practices for 2024
Bohdan / October 31, 2024
Mastering Security in PHP: Best Practices for 2024
With PHP powering a large percentage of web applications worldwide, securing PHP applications is essential. This guide covers advanced techniques and best practices to secure your PHP application from common vulnerabilities, ensuring the integrity, availability, and confidentiality of data.
Table of Contents
- Introduction
- Input Validation and Sanitization
- SQL Injection Prevention
- Cross-Site Scripting (XSS)
- Cross-Site Request Forgery (CSRF)
- Session Management and Security
- File Upload Security
- Data Encryption and Secure Storage
- Error Handling and Logging
- Security Headers
- Regular Security Audits
- Conclusion
Introduction
PHP remains a popular choice for web development, but it’s crucial to secure PHP applications against emerging threats. From input validation to session management, this guide will provide you with a comprehensive set of practices to mitigate security risks.
Input Validation and Sanitization
Proper input validation is one of the first lines of defense against security threats. By validating and sanitizing all user inputs, you can prevent various attacks, such as SQL injection and XSS.
Examples of Input Validation and Sanitization
Use filter_var
and filter_input
to validate user inputs. Here’s an example:
php
$email = filter_input(INPUT_POST, 'email', FILTER_VALIDATE_EMAIL);
if (!$email) {
die("Invalid email format.");
}
$username = htmlspecialchars($_POST['username'], ENT_QUOTES, 'UTF-8');
FILTER_VALIDATE_EMAIL: Ensures email format is correct. htmlspecialchars: Encodes HTML characters, preventing XSS attacks.
SQL Injection Prevention
SQL Injection is a severe vulnerability that allows attackers to manipulate SQL queries by injecting malicious inputs. Prepared statements and parameterized queries prevent SQL injection effectively. Example Using PDO for SQL Injection Prevention
php
$pdo = new PDO('mysql:host=localhost;dbname=test', $user, $pass);
$stmt = $pdo->prepare("SELECT * FROM users WHERE email = :email");
$stmt->execute(['email' => $email]);
Explanation: The use of prepare and execute separates data from the SQL statement, making it safe from injection. Cross-Site Scripting (XSS)
XSS allows attackers to inject malicious scripts into web pages viewed by other users. To prevent XSS, sanitize and encode all user inputs and outputs. Example of XSS Prevention
php
// Sanitize user inputs
$comment = htmlspecialchars($_POST['comment'], ENT_QUOTES, 'UTF-8');
// Output in HTML
echo "<div>$comment</div>";
htmlspecialchars encodes HTML tags, so any script tags are rendered as plain text.
Cross-Site Request Forgery (CSRF)
CSRF forces authenticated users to perform unwanted actions on a web application. Use tokens to verify the request’s legitimacy. Implementing CSRF Tokens
Generate a CSRF Token on form creation. Validate the Token when the form is submitted.
php
// Generate token
session_start();
if (empty($_SESSION['csrf_token'])) {
$_SESSION['csrf_token'] = bin2hex(random_bytes(32));
}
// Add token to form
echo '<input type="hidden" name="csrf_token" value="' . $_SESSION['csrf_token'] . '">';
// Validate token on form submission
if (hash_equals($_SESSION['csrf_token'], $_POST['csrf_token'])) {
// Process form data
} else {
die("Invalid CSRF token.");
}
Explanation: The hash_equals function prevents timing attacks, and bin2hex(random_bytes(32)) creates a secure token. Session Management and Security
Sessions are crucial for user authentication, but they are vulnerable to attacks like session fixation and hijacking. Best Practices for Secure Sessions
Use Secure and HttpOnly Cookies:
php
session_set_cookie_params([
'secure' => true,
'httponly' => true,
'samesite' => 'Strict'
]);
session_start();
Regenerate Session IDs to prevent fixation:
php
session_regenerate_id(true);
File Upload Security
File uploads can introduce risks if files are not properly validated and restricted. Secure File Upload Example
php
$allowed_types = ['image/jpeg', 'image/png'];
$file_type = mime_content_type($_FILES['file']['tmp_name']);
if (!in_array($file_type, $allowed_types)) {
die("Invalid file type.");
}
$target_dir = '/var/www/uploads/';
$target_file = $target_dir . basename($_FILES['file']['name']);
// Move and rename uploaded file
move_uploaded_file($_FILES['file']['tmp_name'], $target_file);
Explanation: mime_content_type checks the file type, while renaming and placing files in secure directories reduces access risk. Data Encryption and Secure Storage
Sensitive data should be encrypted both at rest and in transit to protect against unauthorized access. Example of Data Encryption with OpenSSL
php
$data = "Sensitive data";
$key = 'encryption_key';
$encrypted_data = openssl_encrypt($data, 'aes-256-cbc', $key, 0, 'iv_key_here');
Explanation: Use AES-256 encryption with secure key storage and IVs (initialization vectors) to ensure strong encryption. Error Handling and Logging
Error handling prevents critical application data from being exposed, and logging helps detect anomalies in real time. Secure Error Handling Practices
Turn Off Error Display in Production:
php
ini_set('display_errors', '0');
ini_set('log_errors', '1');
ini_set('error_log', '/var/log/php_errors.log');
Custom Error Handling:
php
function handleError($errno, $errstr, $errfile, $errline) {
error_log("Error: [$errno] $errstr on line $errline in file $errfile");
header("HTTP/1.1 500 Internal Server Error");
exit();
}
set_error_handler("handleError");
Security Headers
Implement security headers to add an extra layer of protection against common attacks. Important Security Headers
Content Security Policy (CSP):
php
header("Content-Security-Policy: default-src 'self'");
X-Frame-Options: Prevents clickjacking.
php
header("X-Frame-Options: SAMEORIGIN");
X-Content-Type-Options: Avoids MIME-type sniffing.
php
header("X-Content-Type-Options: nosniff");
Regular Security Audits
Frequent security audits, including penetration testing, are essential to identify vulnerabilities in PHP applications. Use automated tools like OWASP ZAP and Burp Suite to check for common vulnerabilities. Feature Comparison Table
Here is a comparison of different PHP security techniques and their use cases:
Security Measure | Purpose | Example | Implementation | Difficulty |
---|---|---|---|---|
Input Validation | Prevent SQL injection and XSS | filter_input | Basic | Easy |
SQL Injection Prevention | Secure SQL Queries | PDO | Moderate | Medium |
CSRF Protection | Prevent CSRF Attacks | CSRF Tokens | Advanced | Hard |
File Upload Security | Validate file uploads | mime_content_type | Moderate | Medium |
Encryption | Protect data at rest | OpenSSL | Advanced | Hard |
Security Headers | Protect from common exploits | CSP, X-Frame-Options | Basic | Easy |
Error Handling | Prevent info leakage | Custom Handlers | Basic | Easy |
Conclusion
Securing PHP applications requires a layered approach, integrating various techniques such as input validation, secure session management, CSRF protection, and encryption. By following these best practices and conducting regular audits, you can safeguard your application from vulnerabilities, ensuring secure and resilient PHP applications in 2024 and beyond.
Happy coding and stay secure!